Gmail Account Creator Python
It has an attachment.' #The subject line #The body and the attachments for the mail message.attach(MIMEText(mailcontent, 'plain')) #Create SMTP session for sending the mail session = smtplib.SMTP('smtp.gmail.com', 587) #use gmail with port session.starttls #enable security session.login(senderaddress, senderpass) #login with mailid. Source code: Lib/email/generator.py One of the most common tasks is to generate the flat (serialized) version of the email message represented by a message object structure. You will need to do this if you want to send your message via smtplib.SMTP.sendmail or the nntplib module, or print the message on the console. Then use http.client or Python Requests to interact with that API. If your goal is to create spam or ghost accounts - don't. These services are aimed at quality assurance, ie. Testing email services. Turn on the Gmail API. Create a service account with domain-wide authority. Authorize the service account to send emails. Send emails with a Python script.
- Python Send Email With Gmail
- Gmail Account
- Gmail Account Creator Python
- Gmail Account Creator Python App
Source code:Lib/email/generator.py
One of the most common tasks is to generate the flat (serialized) version ofthe email message represented by a message object structure. You will need todo this if you want to send your message via smtplib.SMTP.sendmail()
orthe nntplib
module, or print the message on the console. Taking amessage object structure and producing a serialized representation is the jobof the generator classes.
As with the email.parser
module, you aren’t limited to the functionalityof the bundled generator; you could write one from scratch yourself. Howeverthe bundled generator knows how to generate most email in a standards-compliantway, should handle MIME and non-MIME email messages just fine, and is designedso that the bytes-oriented parsing and generation operations are inverses,assuming the same non-transforming policy
is used for both. Thatis, parsing the serialized byte stream via theBytesParser
class and then regenerating the serializedbyte stream using BytesGenerator
should produce output identical tothe input 1. (On the other hand, using the generator on anEmailMessage
constructed by program may result inchanges to the EmailMessage
object as defaults arefilled in.)
The Generator
class can be used to flatten a message into a text (asopposed to binary) serialized representation, but since Unicode cannotrepresent binary data directly, the message is of necessity transformed intosomething that contains only ASCII characters, using the standard email RFCContent Transfer Encoding techniques for encoding email messages for transportover channels that are not “8 bit clean”.
To accommodate reproducible processing of SMIME-signed messagesGenerator
disables header folding for message parts of typemultipart/signed
and all subparts.
email.generator.
BytesGenerator
(outfp, mangle_from_=None, maxheaderlen=None, *, policy=None)¶Return a BytesGenerator
object that will write any message providedto the flatten()
method, or any surrogateescape encoded text providedto the write()
method, to the file-like objectoutfp.outfp must support a write
method that accepts binary data.
If optional mangle_from_ is True
, put a >
character in front ofany line in the body that starts with the exact string 'From'
, that isFrom
followed by a space at the beginning of a line. mangle_from_defaults to the value of the mangle_from_
setting of the policy (which is True
for thecompat32
policy and False
for all others).mangle_from_ is intended for use when messages are stored in unix mboxformat (see mailbox
and WHY THE CONTENT-LENGTH FORMAT IS BAD).
If maxheaderlen is not None
, refold any header lines that are longerthan maxheaderlen, or if 0
, do not rewrap any headers. Ifmanheaderlen is None
(the default), wrap headers and other messagelines according to the policy settings.
If policy is specified, use that policy to control message generation. Ifpolicy is None
(the default), use the policy associated with theMessage
or EmailMessage
object passed to flatten
to control the message generation. Seeemail.policy
for details on what policy controls.
Changed in version 3.3: Added the policy keyword.
Changed in version 3.6: The default behavior of the mangle_from_and maxheaderlen parameters is to follow the policy.
flatten
(msg, unixfrom=False, linesep=None)¶Print the textual representation of the message object structure rootedat msg to the output file specified when the BytesGenerator
instance was created.
If the policy
option cte_type
is 8bit
(the default), copy any headers in the original parsedmessage that have not been modified to the output with any bytes with thehigh bit set reproduced as in the original, and preserve the non-ASCIIContent-Transfer-Encoding of any body parts that have them.If cte_type
is 7bit
, convert the bytes with the high bit set asneeded using an ASCII-compatible Content-Transfer-Encoding.That is, transform parts with non-ASCIIContent-Transfer-Encoding(Content-Transfer-Encoding: 8bit) to an ASCII compatibleContent-Transfer-Encoding, and encode RFC-invalid non-ASCIIbytes in headers using the MIME unknown-8bit
character set, thusrendering them RFC-compliant.
If unixfrom is True
, print the envelope header delimiter used bythe Unix mailbox format (see mailbox
) before the first of theRFC 5322 headers of the root message object. If the root object hasno envelope header, craft a standard one. The default is False
.Note that for subparts, no envelope header is ever printed.
If linesep is not None
, use it as the separator character betweenall the lines of the flattened message. If linesep is None
(thedefault), use the value specified in the policy.
clone
(fp)¶Return an independent clone of this BytesGenerator
instance withthe exact same option settings, and fp as the new outfp.
write
(s)¶Encode s using the ASCII
codec and the surrogateescape
errorhandler, and pass it to the write method of the outfp passed to theBytesGenerator
’s constructor.
As a convenience, EmailMessage
provides the methodsas_bytes()
and bytes(aMessage)
(a.k.a.__bytes__()
), which simplify the generation ofa serialized binary representation of a message object. For more detail, seeemail.message
.
Because strings cannot represent binary data, the Generator
class mustconvert any binary data in any message it flattens to an ASCII compatibleformat, by converting them to an ASCII compatibleContent-Transfer_Encoding. Using the terminology of the emailRFCs, you can think of this as Generator
serializing to an I/O streamthat is not “8 bit clean”. In other words, most applications will wantto be using BytesGenerator
, and not Generator
.
email.generator.
Generator
(outfp, mangle_from_=None, maxheaderlen=None, *, policy=None)¶Return a Generator
object that will write any message providedto the flatten()
method, or any text provided to the write()
method, to the file-like objectoutfp. outfp must support awrite
method that accepts string data.
If optional mangle_from_ is True
, put a >
character in front ofany line in the body that starts with the exact string 'From'
, that isFrom
followed by a space at the beginning of a line. mangle_from_defaults to the value of the mangle_from_
setting of the policy (which is True
for thecompat32
policy and False
for all others).mangle_from_ is intended for use when messages are stored in unix mboxformat (see mailbox
and WHY THE CONTENT-LENGTH FORMAT IS BAD).
If maxheaderlen is not None
, refold any header lines that are longerthan maxheaderlen, or if 0
, do not rewrap any headers. Ifmanheaderlen is None
(the default), wrap headers and other messagelines according to the policy settings.
If policy is specified, use that policy to control message generation. Ifpolicy is None
(the default), use the policy associated with theMessage
or EmailMessage
object passed to flatten
to control the message generation. Seeemail.policy
for details on what policy controls.
Changed in version 3.6: The default behavior of the mangle_from_and maxheaderlen parameters is to follow the policy.
flatten
(msg, unixfrom=False, linesep=None)¶Print the textual representation of the message object structure rootedat msg to the output file specified when the Generator
instance was created.
If the policy
option cte_type
is 8bit
, generate the message as if the option were set to 7bit
.(This is required because strings cannot represent non-ASCII bytes.)Convert any bytes with the high bit set as needed using anASCII-compatible Content-Transfer-Encoding. That is,transform parts with non-ASCII Content-Transfer-Encoding(Content-Transfer-Encoding: 8bit) to an ASCII compatibleContent-Transfer-Encoding, and encode RFC-invalid non-ASCIIbytes in headers using the MIME unknown-8bit
character set, thusrendering them RFC-compliant.
If unixfrom is True
, print the envelope header delimiter used bythe Unix mailbox format (see mailbox
) before the first of theRFC 5322 headers of the root message object. If the root object hasno envelope header, craft a standard one. The default is False
.Note that for subparts, no envelope header is ever printed.
If linesep is not None
, use it as the separator character betweenall the lines of the flattened message. If linesep is None
(thedefault), use the value specified in the policy.
Changed in version 3.2: Added support for re-encoding 8bit
message bodies, and thelinesep argument.
clone
(fp)¶Return an independent clone of this Generator
instance with theexact same options, and fp as the new outfp.
write
(s)¶Write s to the write method of the outfp passed to theGenerator
’s constructor. This provides just enough file-likeAPI for Generator
instances to be used in the print()
function.
Python Send Email With Gmail
As a convenience, EmailMessage
provides the methodsas_string()
and str(aMessage)
(a.k.a.__str__()
), which simplify the generation ofa formatted string representation of a message object. For more detail, seeemail.message
.
The email.generator
module also provides a derived class,DecodedGenerator
, which is like the Generator
base class,except that non-text parts are not serialized, but are insteadrepresented in the output stream by a string derived from a template filledin with information about the part.
Gmail Account
email.generator.
DecodedGenerator
(outfp, mangle_from_=None, maxheaderlen=None, fmt=None, *, policy=None)¶Act like Generator
, except that for any subpart of the messagepassed to Generator.flatten()
, if the subpart is of main typetext, print the decoded payload of the subpart, and if the maintype is not text, instead of printing it fill in the stringfmt using information from the part and print the resultingfilled-in string.
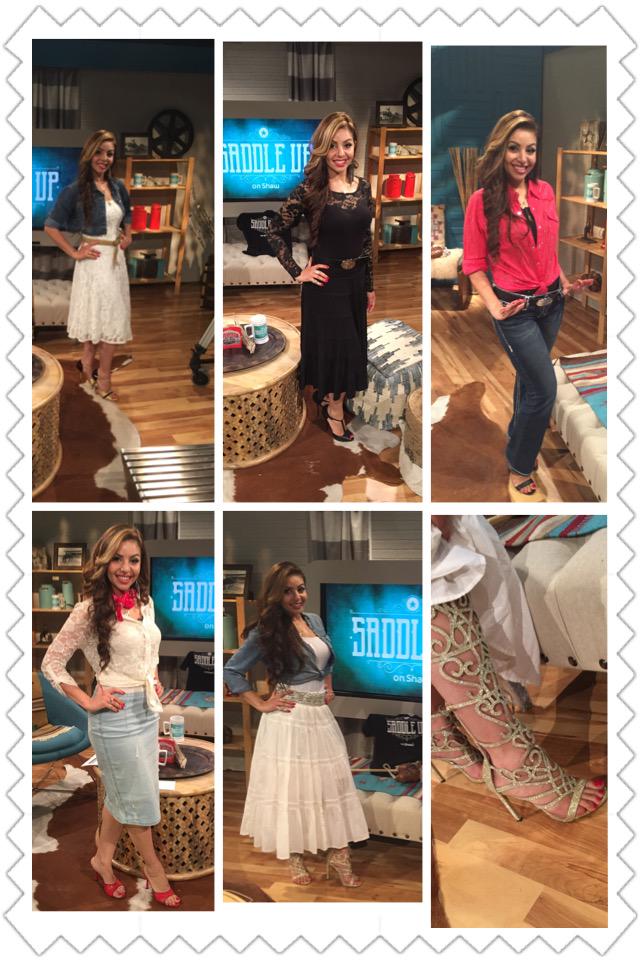
To fill in fmt, execute fmt%part_info
, where part_info
is a dictionary composed of the following keys and values:
type
– Full MIME type of the non-text partmaintype
– Main MIME type of the non-text partsubtype
– Sub-MIME type of the non-text partfilename
– Filename of the non-text partdescription
– Description associated with the non-text partencoding
– Content transfer encoding of the non-text part
If fmt is None
, use the following default fmt:
“[Non-text (%(type)s) part of message omitted, filename %(filename)s]”
Optional _mangle_from_ and maxheaderlen are as with theGenerator
base class.
Gmail Account Creator Python
Footnotes
Gmail Account Creator Python App
This statement assumes that you use the appropriate setting forunixfrom
, and that there are no policy
settings calling forautomatic adjustments (for example,refold_source
must be none
, which isnot the default). It is also not 100% true, since if the messagedoes not conform to the RFC standards occasionally information about theexact original text is lost during parsing error recovery. It is a goalto fix these latter edge cases when possible.